The Anthropic Dice Killer Simulation
Jan 13, 2024
10 min read
views
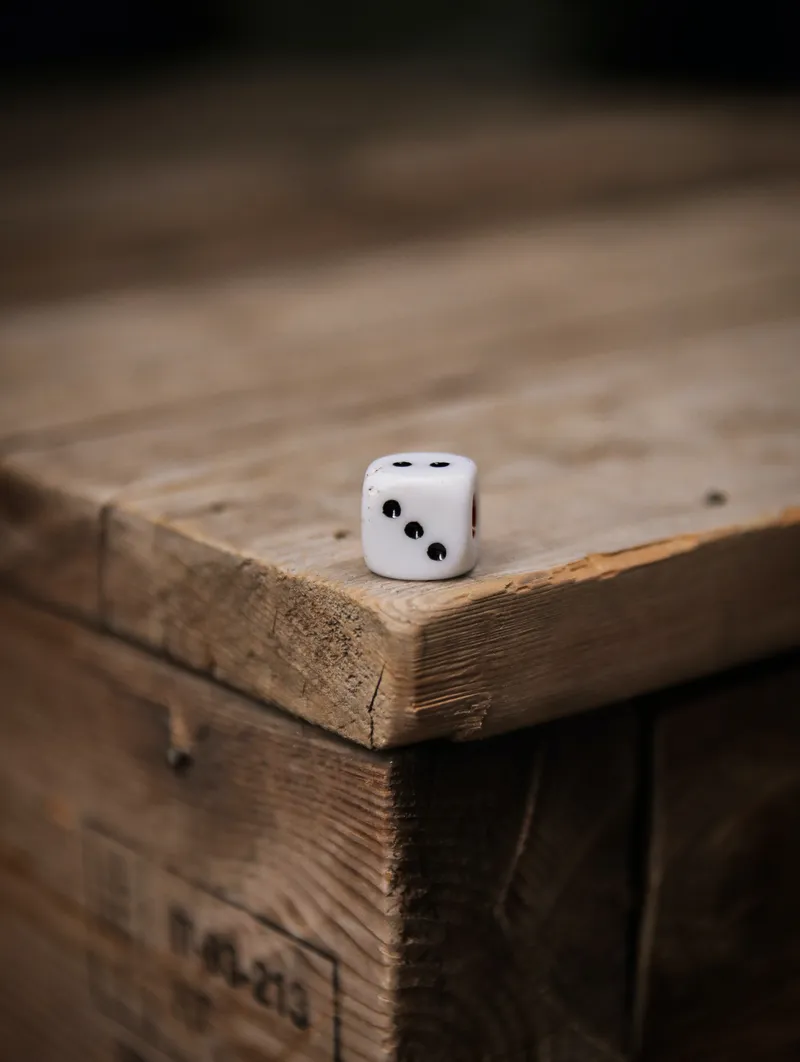
Problem Statement
Imagine that there’s a murderer on the loose. He kidnaps your neighbor, Bob, and blindfolds him. The killer then rolls a pair of dice. If he rolls a “snake eyes” (a 1 on each die), he kills Bob. Otherwise, he releases him and kidnaps 10 random people, and then rolls the dice again. If he rolls a snake eyes, he kills them all. Otherwise, he again releases them and kidnaps 100 more random people. This continues until the killer rolls a snake eyes, at which point he kills all hostages.
Unfortunately, you decide to go for a walk one afternoon and you unknowingly pass by the dice killer’s mansion. Next thing you know, you wake up blindfolded. Luckily, you are able to peek through a gap in the fabric. While the dice killer is blindfolding the other hostages in the room, you see an escape route draped in cobwebs. If you take it, there is a 50% chance that you will die. You can either take the escape route or you can wait to see how the dice roll.
What do you do?
Taking Your Chances with the Dice
The killer is rolling a pair of fair dice with 6 sides each. Rolling Die A has no effect on the result of the Die B, so they are independent of each other. Because of this, we can use the following formula:
So let’s do the quick calculation. For clarity, “die” in the equations below is the singular form of “dice”.
Your chance of death is 1 in 36, which comes out to about ~ 2.78%. If you take the escape route, your chances of death are 1 in 2. So what’s the problem? Aren’t you always better off taking your chances with the dice?
Taking the Escape Route
The case for the escape route is this: If you consider the fraction of people the murderer will kill over the course of his kidnapping spree, you will find that the expected fraction of people the murderer will kill is greater than 50%.
For reference, the “expected value” of a distribution is a statistical average. The formula for calculating the expected value of a distribution “X” is:
Effectively, to find the expected value, you sum all possible outcomes and weight each outcome by its probability.
- Let f be the fraction of kidnapped people who are murdered.
- In round 1, there is a 1 in 36 chance that 1 person is killed. There is only 1 person kidnapped. We expect 1/36th of a person to be killed in this round.
- There’s a 35 in 36 chance we get to round 2. Once there, there’s a 1 in 36 chance that 10 people are killed. We expect 5/18ths of a person to be killed. There have been 11 people kidnapped if we get to this round.
- The chance of getting to round 3 is 35^2/36^2. If we get there, we should expect 100/36 (almost 3) people to be killed in this round after 111 kidnappings.
- We continue this sequence to infinity (or until the killer has kidnapped the entire population).
- From the first set of terms, it may seem that the fraction of people murdered to people kidnapped is quite low. However, consider that we should expect (on average) 36 rounds of kidnapping. Also consider that with each round, we have 10 times as many people kidnapped. When the murderer finally rolls a snake eyes, the number of people murdered in the current round is always going to be greater than the sum of all people kidnapped from previous rounds.
This generalizes to the following:
We can approximate the expected value by calculating the sum of the first 5000 terms. The “5000” here is arbitrary; we could pick any large number because what we are trying to do is approximate the limit as the number of dice rolls approaches infinity. To do this, we can use Python. (We are using Python here since it’s more readable than many general purpose programming languages out there.)
A few things to note about the below snippet:
- It defines a Python function that generalizes this problem a little further.
- Type hints are included to make it clearer what is an integer and what is a decimal.
- Feel free to run this on your own machine!
def get_expected_fraction_of_people_murderered(
growth_factor: int, # 10 in this case, since the killer will kidnap 10 times as many people after each dice roll.
first_n_terms: int, # An arbitrarily high positive integer
probability_of_hostages_dying: float # The chance of the hostages dying in each round.
) -> float:
fraction_of_people_murdered: float = 0.0 # This will be an approximation that gets closer as "first_n_terms" increases.
probability_of_hostages_being_released: float = 1 - probability_of_hostages_dying # = 35/36 if the chance of rolling snake eyes is 1/36
for i in range(1, first_n_terms + 1): # Because range goes from [start, end), we have to increment "first_n_terms" here so it goes, for example, i = 1, i = 2, i = 3 if first_n_terms = 3.
first_operand = probability_of_hostages_dying * (probability_of_hostages_being_released ** (i - 1))
second_operand_divisor = 0
for j in range(0, i):
second_operand_divisor += growth_factor ** j
second_operand = (growth_factor ** (i - 1)) / second_operand_divisor
fraction_of_people_murdered += first_operand * second_operand
return fraction_of_people_murdered
print(get_expected_fraction_of_people_murderered(10, 5000, (1 / 36))) # ~ 0.903049487539702
Approximately 90% of the hostages will unfortunately be killed.
Does this change your decision about whether to take the escape route?
The Answer
There appears to be a paradox here. However, unlike many thought experiments, sticking to your intuitions and letting the dice roll how they may is the correct decision.
Here’s why.
You are only kidnapped in a single round. The implicit (but key) assumption is that once kidnapped, you cannot be kidnapped again in a later round. Despite the fact that 90% of those kidnapped will die, it is still rational to let the dice roll and not take the escape route because a 35 in 36 chance of being released is better than a 1 in 2 chance of successfully taking the escape route. Obviously, right? The reason the percentage of people killed is so high is only because of the final round. You are dealing with a conditional probability. Given that you are kidnapped and blindfolded in only a single round, you must decide between taking the escape route and letting the dice roll. It works out that letting the dice roll is the best option for the intuitive reason.
How to Get the Opposite Answer
However, let’s imagine a slightly different scenario.
Imagine that you already live in the same town as the killer. The only way to avoid being kidnapped is to move to another town, but the only highway that connects the 2 cities is very, very dangerous. It’s replete with robbers, crooks, and criminals of every kind. Only half of all travelers actually make it to their destination alive.
If you decide to stay and take your chances with the dice killer, there is no escape route once kidnapped (since it wouldn’t be rational to ever choose this anyway). Moreover, if the dice killer releases you, it is now possible for the killer to kidnap you again later. The dice killer (just as before) stops the first time he rolls a snake eyes.
The question is: If you had to pick between moving to another town or taking your chances with being kidnapped, which should you choose?
Take a second to think about your answer.
Admittedly, I misled you a bit here. As it turns out, you still don’t know enough about the town to say which is the better option because you don’t know the expected fraction of the population that will be kidnapped. If the town has only 500 inhabitants, you are better off driving the dangerous highway. If the town has a population of 10 ^ 100, then you are better off staying in the town with the dice killer because of how unlikely it is that you will be kidnapped. You’d need to know the population of the town to say, but with a reasonably-sized town or city, you should definitely pack your bags, put your house up for sale, and book it to the neighboring town.
The tipping point for the population of the dice killer’s town (i.e., where the decision tree splits) depends on a couple of variables: the probability the killer executes the hostages in each round (e.g., 1 in 36) and the growth factor between each round. Unfortunately, changing these variables would also change the fraction of hostages who are killed, so we’d need to rerun the Python function defined earlier with different arguments. In addition, we might also need to account for when the dice killer is tasked with kidnapping more people than there are people in the town. To make that concrete, what if the dice killer is supposed to kidnap 100 people and the population of the town is only 75? In short, once we account for the town population and the edge cases, we can find the optimal survival strategy.
Simulator
Your objective is to survive.
There are 2 ways to survive. You can either travel the dangerous road without perishing or you can hide until the number of hostages exceeds the population.
Conclusion
Hope you never find yourself in as dire a situation as with the dice killer, but hopefully this unwinds the paradox for you and gives you the statistical tools to think through similar problems (and potentially real-world problems, as well).
References
This post draws heavily upon the blog post from Rising Entropy, so credit to the author for laying out the problem that is restated and (to some extent) reanalyzed here.